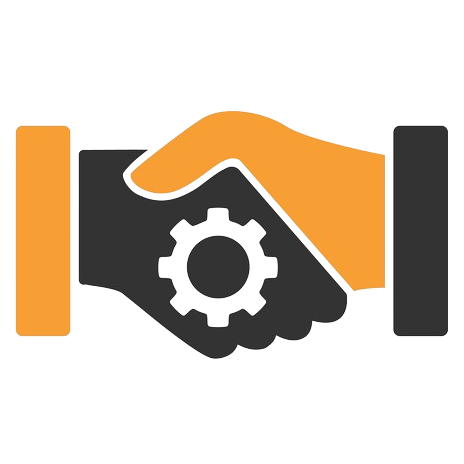
What are Smart Contracts?
In recent years, the advent of blockchain technology has sparked a wave of innovation across various industries, and one of the most exciting developments to emerge from this technological revolution is the concept of smart contracts. Smart contracts have the potential to transform traditional business processes by automating agreements, reducing costs, enhancing transparency, and increasing efficiency. In this article, we will explore what smart contracts are, how they work, and their potential applications in various sectors.
Smart contracts are self-executing contracts with the terms of the agreement directly written into computer code. They are stored on a blockchain, which is a decentralized and distributed digital ledger that is secured by cryptography. The blockchain ensures that smart contracts are tamper-proof and transparent, as every transaction and contract execution is recorded and verified by the network of computers participating in the blockchain.
One of the key features of smart contracts is their ability to operate autonomously, without the need for intermediaries. Traditional contracts often require the involvement of third parties, such as lawyers or notaries, to ensure trust and enforceability. Smart contracts eliminate the need for these intermediaries, as the code itself enforces the terms of the agreement. This not only streamlines the contracting process but also reduces costs and eliminates the potential for human error or bias.
Smart contracts can be used in a wide range of applications across various industries. For example, in the financial industry, smart contracts can be used for automated payment processing, digital identity verification, and lending and borrowing agreements. In supply chain management, smart contracts can be used to track and verify the movement of goods, ensure compliance with regulations, and automate payments. In the real estate industry, smart contracts can be used for property transfers, rental agreements, and property management. Smart contracts can also be used in areas such as insurance, healthcare, intellectual property, and voting systems, among others.
One of the main benefits of smart contracts is their transparency. As all transactions and contract executions are recorded on the blockchain, they can be easily audited and verified. This helps to prevent fraud, corruption, and manipulation, as the data on the blockchain is immutable and cannot be altered. This transparency also promotes trust among parties, as all participants can access and verify the details of the smart contract, eliminating the need to rely solely on trust in a counterparty.
Another advantage of smart contracts is their efficiency. Traditional contracts often require time-consuming and manual processes for execution, verification, and enforcement. Smart contracts, on the other hand, are automated and self-executing, reducing the need for human intervention and increasing the speed of contract execution. This can lead to significant cost savings and faster business processes.
However, it's important to note that smart contracts are not without challenges and limitations. One of the main challenges is the lack of legal clarity and regulation surrounding smart contracts. As smart contracts are still a relatively new technology, there are legal and regulatory issues that need to be addressed, such as contract validity, liability, and dispute resolution. Another challenge is the potential for coding errors or vulnerabilities in the smart contract code, which could lead to unintended consequences or security breaches.
In conclusion, smart contracts are a promising development in the field of blockchain technology that have the potential to revolutionize traditional business processes. They offer advantages such as automation, transparency, and efficiency, and can be used in various industries and applications. However, challenges related to legal and regulatory frameworks, as well as the potential for coding errors, need to be addressed to ensure the widespread adoption and successful implementation of smart contracts in the future. As the technology continues to evolve, smart contracts are likely to play a significant role in shaping the way businesses and industries operate in the digital age.
Example
Below is an example of a smart contract written for the Ethereum Virtual Machine. It creates fungible tokens which can be used by applications and their users for permissionless, tokenized transactions.
// SPDX-License-Identifier: MIT
pragma solidity ^0.8.17;
// https://github.com/OpenZeppelin/openzeppelin-contracts/blob/v3.0.0/contracts/token/ERC20/IERC20.sol
interface IERC20 {
function totalSupply() external view returns (uint);
function balanceOf(address account) external view returns (uint);
function transfer(address recipient, uint amount) external returns (bool);
function allowance(address owner, address spender) external view returns (uint);
function approve(address spender, uint amount) external returns (bool);
function transferFrom(
address sender,
address recipient,
uint amount
) external returns (bool);
event Transfer(address indexed from, address indexed to, uint value);
event Approval(address indexed owner, address indexed spender, uint value);
}
// SPDX-License-Identifier: MIT
pragma solidity ^0.8.17;
import "./IERC20.sol";
contract ERC20 is IERC20 {
uint public totalSupply;
mapping(address => uint) public balanceOf;
mapping(address => mapping(address => uint)) public allowance;
string public name = "Solidity by Example";
string public symbol = "SOLBYEX";
uint8 public decimals = 18;
function transfer(address recipient, uint amount) external returns (bool) {
balanceOf[msg.sender] -= amount;
balanceOf[recipient] += amount;
emit Transfer(msg.sender, recipient, amount);
return true;
}
function approve(address spender, uint amount) external returns (bool) {
allowance[msg.sender][spender] = amount;
emit Approval(msg.sender, spender, amount);
return true;
}
function transferFrom(
address sender,
address recipient,
uint amount
) external returns (bool) {
allowance[sender][msg.sender] -= amount;
balanceOf[sender] -= amount;
balanceOf[recipient] += amount;
emit Transfer(sender, recipient, amount);
return true;
}
function mint(uint amount) external {
balanceOf[msg.sender] += amount;
totalSupply += amount;
emit Transfer(address(0), msg.sender, amount);
}
function burn(uint amount) external {
balanceOf[msg.sender] -= amount;
totalSupply -= amount;
emit Transfer(msg.sender, address(0), amount);
}
}